Correlated random numbers in R
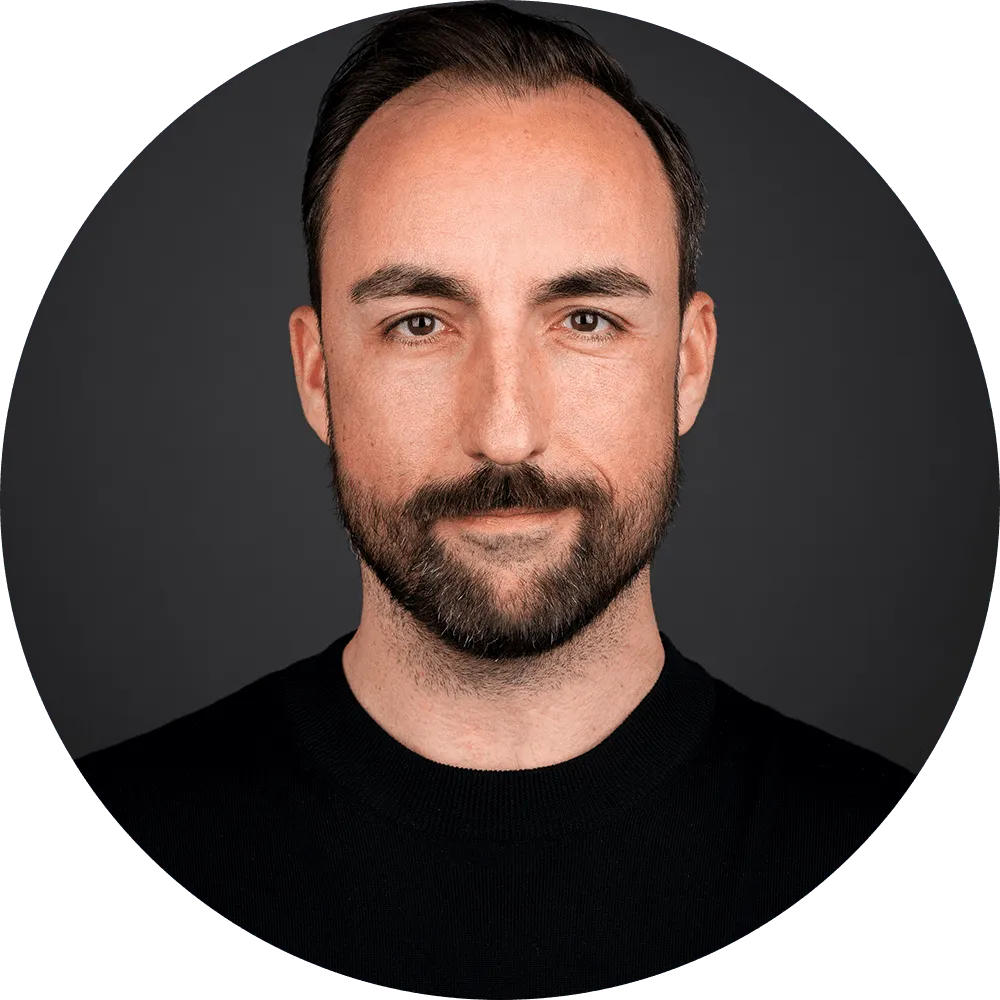

Generating correlated random variables is one of the key aspects of programming simulations. Stock returns, time series, MCMC algorithms, and many other methods can be used by creating correlated random numbers.
Decomposing the Correlation Matrix
Correlated random numbers can be generated by multiplying the n x p matrix of random values with the desired Cholesky-decomposed correlation matrix C of the random values. A Cholesky decomposition can easily be performed in R using the chol() function. This approach is applied, for example, in simulating correlated time series developments in finance (e.g., stock price movements). In our example, we simulate the returns of two stocks and visualize them in an X-Y scatter plot along with the corresponding linear fit. To do this, we use a feature in RStudio that allows for the creation of simple, interactive plots. This requires the R package manipulate, which is installed along with RStudio.
Interactive Plots in RStudio with Manipulate
manipulate() generates sliders or checkboxes that allow the user to vary plot parameters. In our example, the following parameters can be adjusted by the user:
- Correlation of random values r
- Number of random returns n
- Mean returns m1 and m2
- Standard deviation of returns s1 and s2
At the beginning of the code, R’s random number generator "seed" is fixed so that the same random numbers are generated when the code is re-executed. This is done using the set.seed() function. After running the code, a small gear icon appears in the upper left corner of RStudio’s plot window. Clicking on it displays the interactive parameters, which can now be adjusted. The plot automatically updates according to the selected parameter. The code can be copied and pasted directly into RStudio. Have fun experimenting!
# Loading libraries
library(manipulate)
library(ggplot2)
# Seed
set.seed(123)
# Interactive chart to visualise correlated stock returns
manipulate(
# Plot
qplot(x=X1, y=X2, xlab="Rendite Aktie X", ylab="Rendite Aktie Y",
data=data.frame(matrix(nrow=n, ncol=2,
data=cbind(rnorm(n, m1, s1), rnorm(n, m1, s1))) %*%
chol(matrix(nrow=2, ncol=2, data=c(1, r, r, 1))))) +
theme_bw() +
stat_smooth(method="lm"),
# Slider
r = slider(-0.99, 0.99),
n = slider(100, 1000),
m1 = slider(0, 0.1),
s1 = slider(0.01, 0.05),
m2 = slider(0, 0.1),
s2 = slider(0.01, 0.05)
) # Ende manipulate
Summary
With manipulate(), interactive visualizations can be created quickly. For larger projects in the field of interactive visualization, Shiny - the HTML5/CSS/JavaScript framework from RStudio - is more suitable. It allows you to develop complex applications that can be published on an intranet or the internet. If you have any questions about interactive visualization, our experts are happy to assist you at info@statworx.com.