Error handling in R - Handling exceptions with trycatch
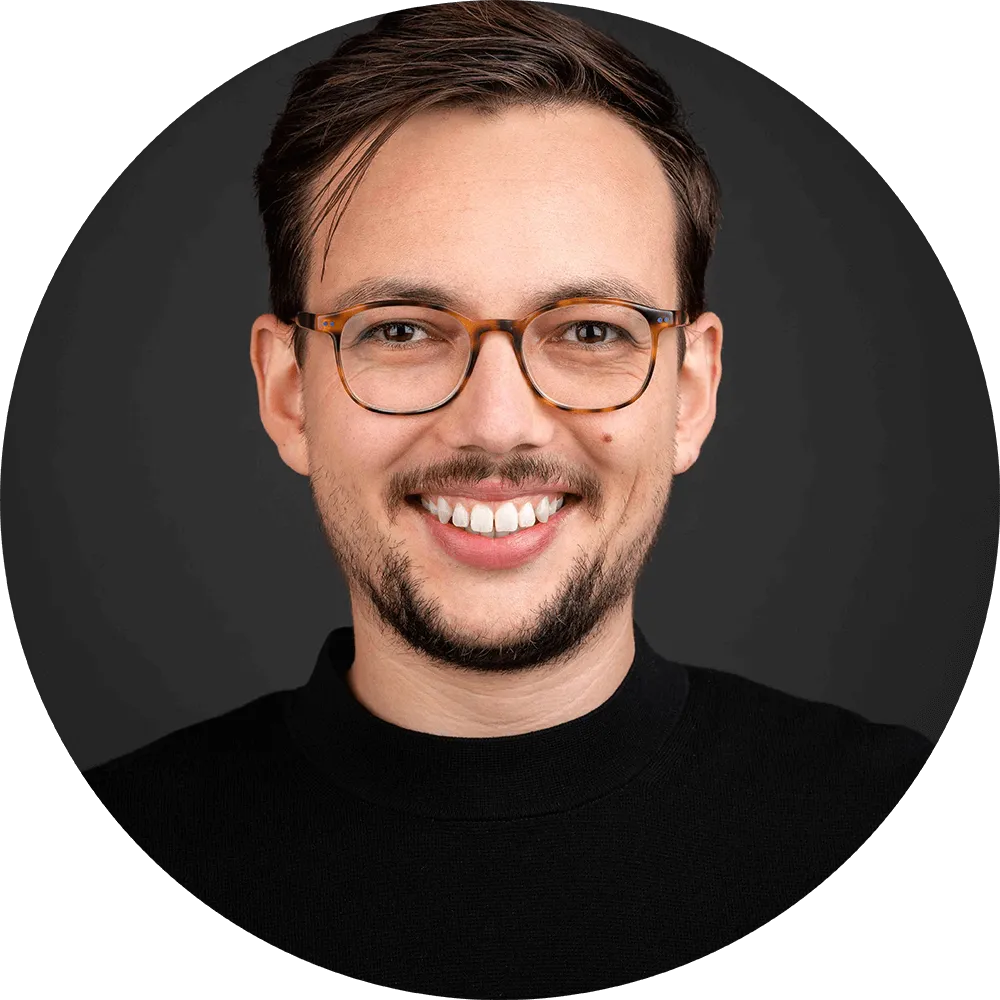

The previous part of the series focused on handling unexpected errors and bugs. However, sometimes errors are expected, for example, when applying the same model to multiple datasets. One possible error in this scenario is that the model cannot be estimated due to a lack of variance. In such cases, one does not want the entire estimation process to stop because of a single error but instead wants the next estimation to proceed.
In R, there are three different methods to achieve this:
try()
ignores the error and continues the calculation.tryCatch()
allows assigning an additional error message or action.withCallingHandlers()
is a special variant oftryCatch()
, which handles exceptions locally. Since it is rarely needed, we will focus on the first two methods.
try
With try()
, the code continues execution regardless of any occurring errors. In the following example, an error occurs, causing the process to terminate, and no value is returned.

If we wrap the error-generating function in a try()
block, the error message is still displayed, but the rest of the code continues executing, and a return value is still provided.

By activating the option silent = TRUE
within the try()
function, the error message is no longer displayed at all. Code blocks are enclosed within {}
inside try()
.
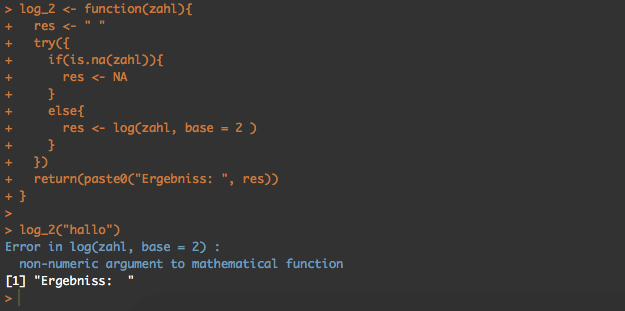
Additionally, it is possible to check the class of try()
. If no error occurs, it returns the class of the last return value; however, if an error occurs, it returns a special "try-error"
class.

This allows for a subsequent check of whether the function was executed successfully, which is particularly useful when applying a function to multiple objects.
trycatch
Unlike try()
, tryCatch()
can handle not only errors but also warnings, messages, and interruptions. Another key feature is that different functions can be executed depending on the type of exception encountered. Typically, default values are assigned, or more meaningful messages are generated. In the following example, we loop through a vector with the values data.frame(4, 2, -3, 10, "hello")
. The log
function produces a warning for negative values and an error for factors and strings.
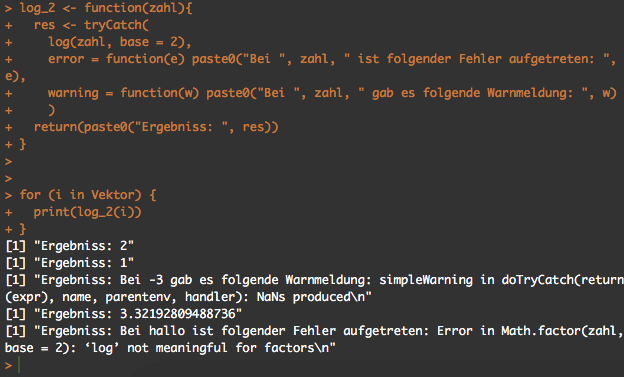
The last important argument of tryCatch()
is finally
. The function specified there is executed last, regardless of whether the preceding code ran successfully or was interrupted. This is useful for deleting unnecessary objects or closing connections. The next article in this series will focus on unit testing.
References
- Advanced R by Hadley Wickham